Most web service platforms provide us mechanism for creating an object that can be used to communicate with the service... those objects are called proxies.WCF provides us several mechanisms for creating proxy objects that can be used to communicate with service.
Generating Proxy classes from service metadata:-
There are two ways to create proxies using metadata.
1.Using Svcutil.exe
2.Using Visual Studio to Generate proxy
.Net 3.0 Framework provides a command line utility called svcutil that we can use for generating proxies;location of this utility is...
..\Program Files\Microsoft SDKs\Windows\v6.0\Bin
The following is an example how you might use the utility...
Svcutil http://localhost:8080/WeatherService.svc /out: ServiceProxy.cs /config: app.config
Couple of things about command example
1. C# is default language for other language you need to provide language option
2. Out is for output option and config is for configuration file generation. Other options can be found at following location
http://msdn.microsoft.com/en-us/library/aa347733.aspx
Visual studio to generate Proxy:
In visual studio you can add service reference by right clicking project node in solution explorer and choosing Add service reference...
In the resulting dialog box enter the endpoint address of service endpoint and click Go to discover to browse for available service... click ok to generate proxy
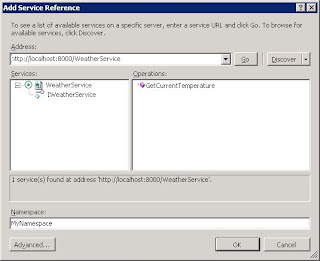
[Service Reference:-2]
Manually creating Proxy class:-
Opposed to having a tool to generate a proxy class we can manually define our own proxy class using ‘ClientBase’ as base class tools also use this call behind the scenes...
e.g.
[ServiceContract]
Interface IWeatherService
{
[OperationContract]
CurrentTemperatureResponse GetCurrentTemperature ();
}
We could manually define proxy class based on that contract as follows...
Public Class WeatherServiceProxy : ClientBase< IWeatherService >, IWeatherService
{
Public WeatherServiceProxy (Binding binding, EndpointAddress epAddr)
{
}
Public WeatherServiceProxy (string endpointConfName)
: Base (endpointConfName)
{
}
Public CurrentTemperatureResponse GetCurrentTemperature ()
{
return this.Channel.GetCurrentTemperature ();
}
}
Dynamically Creating Proxy:-
In few cases we do not need to have proxy class explicitly define anywhere because WCF provides the ChannelFactory class as a mean of creating proxy objects based on Service Contract alone. The following code shows how this would be done....
Binding objBinding = new WSHttpBinding ();
ChannelFactory< IWeatherService > Factory =;
Factory = new ChannelFactory< IWeatherService >
(objBinding, “http://localhost:8080/WeatherService.svc”);
Try
{
IWeatherService Proxy = Factory.CreateChannel ();
CurrentTemperatureResponse argRes = new CurrentTemperatureResponse ();
argRes = Proxy. GetCurrentTemperature ();
}
Catch (System.ServiceModel.FaultException ex)
{
Messagebox.show (ex.FaultReason);
}
So above are the ways to create proxy object we use them according to projects need.
Best Practices:-
One of the principles of service oriented development client should only on service’s schema and not any on service’s class... So the best practice to consume service is through service’s metadata... if manually or dynamically creating service proxy then we need to have access to WCF contracts; in that case Contracts binary files have to be shard by client and service and we should avoid to form this type of strict coupling....